
JExcel
Developer(s) | TeamDev |
---|---|
Stable release | 1.7
|
Written in | Java |
Operating system | Cross-platform |
Type | API to access Microsoft Excel format |
License | TeamDev[1] |
Website | https://www.teamdev.com/jexcel |
JExcel is a library (API) to read, write, display, and modify Excel files with .xls or .xlsx formats. API can be embedded with Java Swing and AWT. [2][3][4]

JExcel support is discontinued as of May 31, 2020.[5]

Some features
Some main features are as follows:

- Automate Excel application, workbooks, spreadsheets, etc.
- Embed workbooks in a Java Swing application as ordinary Swing component
- Add event listeners to workbooks and spreadsheets
- Add event handlers to handle the behavior of workbook and spreadsheet events
- Add native peers to develop custom functionality.[2][3][4]
Usage
Primary usage is handling Excel files through its API.
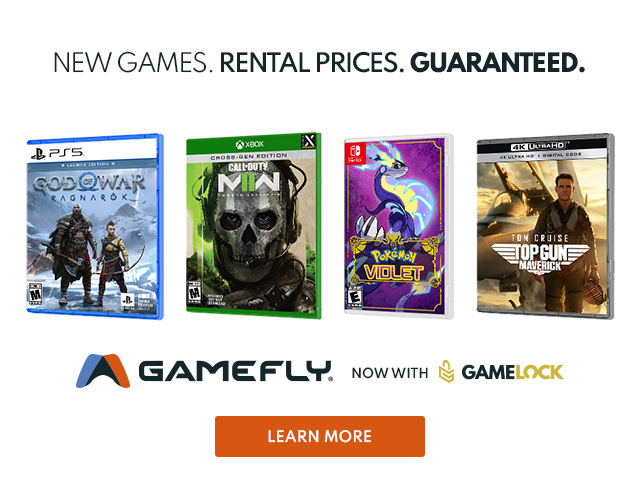
Example
Sample code for reading/writing workbook attributes, setting password, and saving MS Excel 2003 format, might look like as follows:

import com.jniwrapper.win32.jexcel.Application;
import com.jniwrapper.win32.jexcel.FileFormat;
import com.jniwrapper.win32.jexcel.GenericWorkbook;
import com.jniwrapper.win32.jexcel.Workbook;
import java.io.File;
/**
* This sample shows how to read/modify workbook attributes, how to save workbook in Excel 2003 format,
* and how to reopen workbook.
*
* The sample works with MS Excel in non-embedded mode.
*/
public class WorkbookSample
{
public static void main(String[] args) throws Exception
{
//Start MS Excel application, crate workbook and make it visible.
// Application starts invisible and without any workbooks
Application application = new Application();
Workbook workbook = application.createWorkbook("Custom title");
printWorkbookAttributes(workbook);
modifyWorkbookAttributes(workbook);
File newFile = new File("Workbook.xls");
//Save workbook in Excel 2003, to save in Excel 2007 format use FileFormat.OPENXMLWORKBOOK
// format specificator and *.xlsx extension
workbook.saveAs(newFile, FileFormat.WORKBOOKNORMAL, true);
File workbookCopy = new File("WorkbookCopy.xls");
workbook.saveCopyAs(workbookCopy);
//Close workbook saving changes
workbook.close(true);
//Reopening the workbook
workbook = application.openWorkbook(newFile, true, "xxx001");
printWorkbookAttributes(workbook);
//Perform cleanup after yourself and close the MS Excel application forcing it to quit
application.close(true);
}
/**
* Prints workbook attributes to console
* @param workbook - workbook to print information about
*/
public static void printWorkbookAttributes(GenericWorkbook workbook)
{
String fileName = workbook.getFile().getAbsolutePath();
String name = workbook.getWorkbookName();
String title = workbook.getTitle();
String author = workbook.getAuthor();
System.out.println("\n[Workbook Information]");
System.out.println("File path: " + fileName);
System.out.println("Name: " + name);
System.out.println("Title: " + title);
System.out.println("Author: " + author);
if (workbook.hasPassword())
{
System.out.println("The workbook is protected with a password");
}
else
{
System.out.println("The workbook is not protected with a password");
}
if (workbook.isReadOnly())
{
System.out.println("Read only mode");
}
}
/**
* Modify workbook title, author and set password
* @param workbook - workbook to modify attributes
*/
public static void modifyWorkbookAttributes(GenericWorkbook workbook)
{
workbook.setTitle("X-files");
workbook.setPassword("xxx001");
workbook.setAuthor("Agent Smith");
}
}

See also
References
- ^ "JExcel Product Licence Agreement". TeamDev. TeamDev. Retrieved 2 February 2016.
- ^ a b "recall". Recall. Archived from the original on 2 February 2016. Retrieved 2 February 2016.
- ^ a b "JExcel 1.7". GearDownload. GearDownload. Retrieved 2 February 2016.
- ^ a b "JExcel 1.7". Directory of Shareware. Directory of Shareware. Retrieved 2 February 2016.
- ^ "JExcel Product Website". TeamDev. TeamDev.
- ^ "Reading\writing workbook attributes. Setting password. Saving workbook in MS Excel 2003 format". JExcel Support. JExcel Support. Retrieved 2 February 2016.
External links
- JExcel – the official JExcel page.
- JExcel Support - the JExcel Support website containing documentation, release notes and examples.
See what we do next...
OR
By submitting your email or phone number, you're giving mschf permission to send you email and/or recurring marketing texts. Data rates may apply. Text stop to cancel, help for help.
Success: You're subscribed now !